Access application files on iOS simulator
During development we often need to check what files are produced by our application. Sometimes we need to add some files to our app folder for testing. In this article we will see how we can access and manage application files on the iOS simulator.
# Get path to app directory
To get the path to our application directory on the simulator, we will add a print statement inside application(_:didFinishLaunchingWithOptions:)
method in AppDelegate
class. This will print NSHomeDirectory()
in Xcode console on app launch.
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
print("Application directory: \(NSHomeDirectory())")
return true
}
}
# Open application folder in Finder
First, copy the path to the app folder from Xcode console.
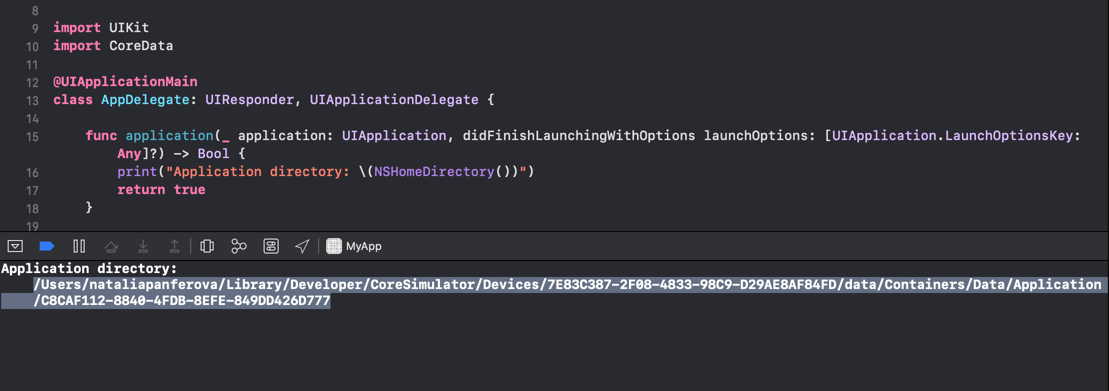
Then open Finder, click on Go -> Go to Folder and paste the application directory path.
You will now be able to browse all the files in your application folder.
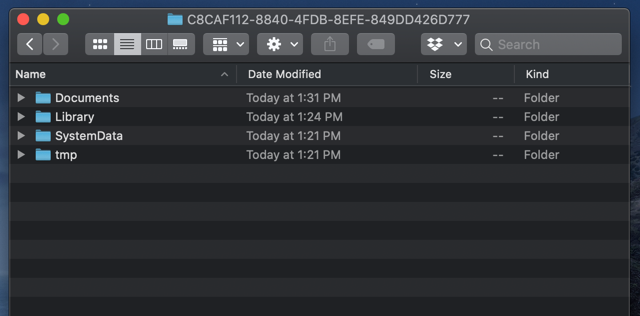
# Manage files in Documents folder
Files that your app saves in .documentDirectory
will appear inside the Documents
folder. You can open them to check their contents or you can add some extra files for testing.
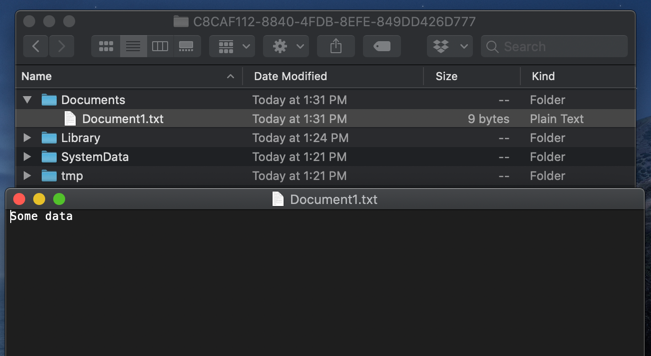
# Browse Core Data SQLite database
If your app uses Core Data, you can find your database .sqlite
file inside Library/Application Support
. You can then use any SQLite viewer to browse the contents of your database, for example SQLPro for SQLite.