Getting ready for iOS 14 local network privacy restrictions
Apple added privacy control in iOS 14 that affects apps accessing the local network. When your app tries to access the user's local network, iOS will show a prompt asking the user to allow access. This should be tested on a real device.
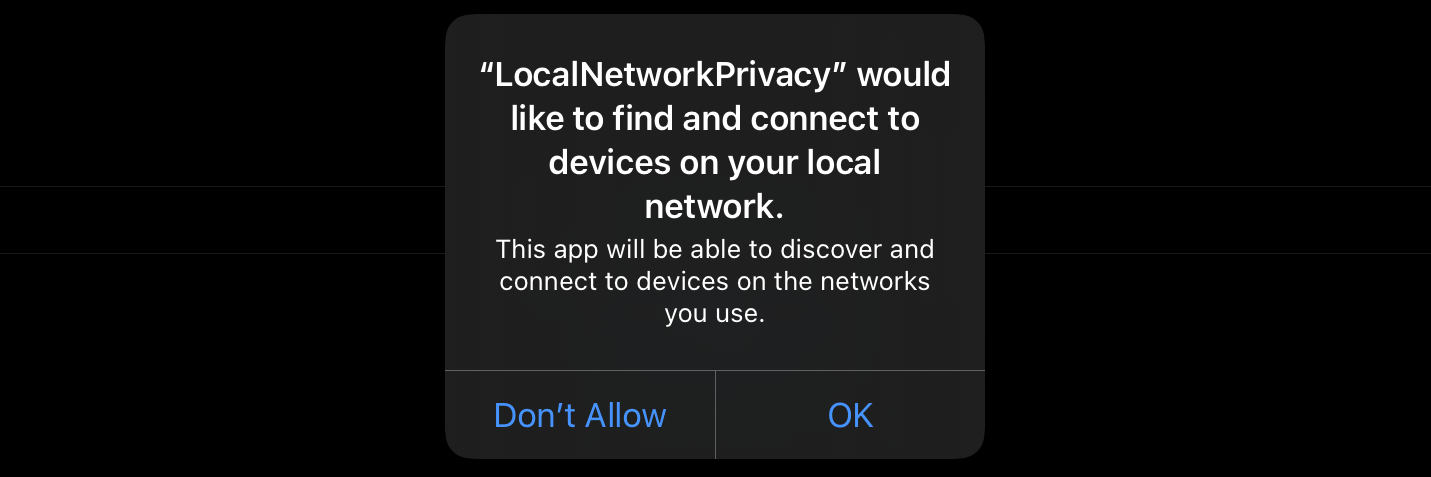
We can customize the usage description in the Info.plist
file using the key NSLocalNetworkUsageDescription
.
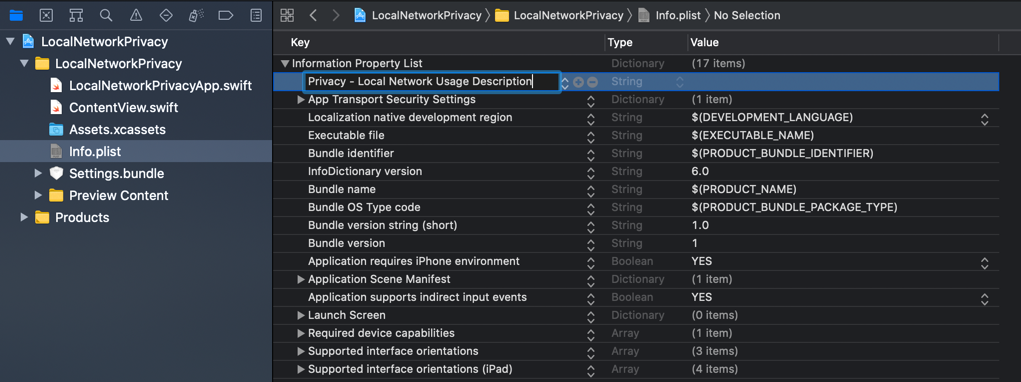
If the user doesn't allow access, the app won't be able to connect to devices on local network. In our example, if the user tries to connect to a local HTTP server, we'll get an error The Internet connection appears to be offline
.
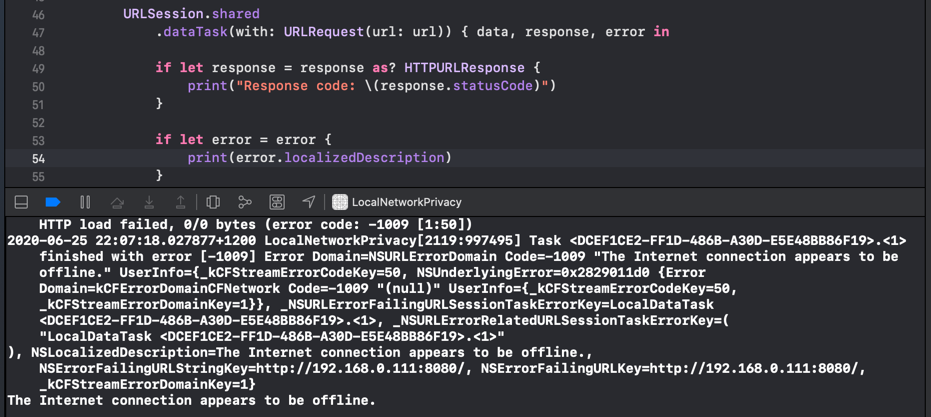
If the user allows access, then the request should go through once permission is granted. For this we will need to create URLSession
with waitsForConnectivity
set to true
in configuration and an appropriate timeout.
let sessionConfig = URLSessionConfiguration.default
sessionConfig.waitsForConnectivity = true
sessionConfig.timeoutIntervalForResource = 60
URLSession(configuration: sessionConfig)
.dataTask(with: URLRequest(url: url)) { data, response, error in
if let response = response as? HTTPURLResponse {
print("Response code: \(response.statusCode)")
}
if let error = error {
print(error.localizedDescription)
}
}.resume()
The user can change the Local Network access permissions inside the device Settings for your app.
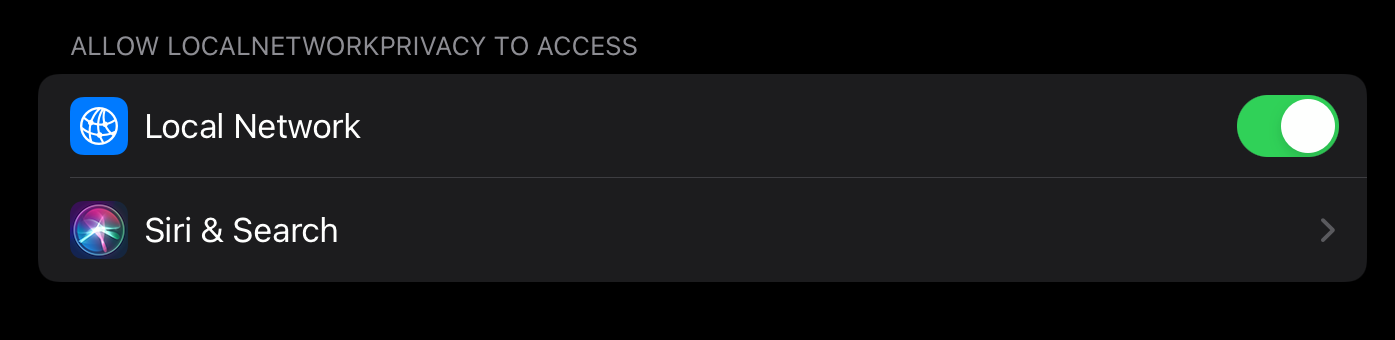