Handle plurals in SwiftUI Text views with inflection
The Foundation framework has a feature called automatic grammar agreement that reduces the number of localized strings we need to provide while making our code simpler. It ensures text follows grammatical rules like pluralization and gender. It works seamlessly with SwiftUI, allowing us to handle plurals directly in Text views without any extra manual logic.
To make text automatically adjust to plural values, we can specify that we want it to use the inflection rule and define the scope for the inflection using the following syntax:
Text("You read ^[\(bookCount) book](inflect: true) this year!")
SwiftUI recognizes this syntax as a custom Markdown attribute and processes it accordingly. When a Text view is created with a string literal, as shown in the example above, SwiftUI treats the string as a LocalizedStringKey
and parses the Markdown it contains. It identifies inflection attributes within the string and uses the automatic grammar agreement feature from Foundation to apply the necessary adjustments during rendering.
Here’s how that would look in a complete SwiftUI view:
struct BookReadingTracker: View {
@State private var bookCount: Int = 0
var body: some View {
VStack(spacing: 16) {
Text("""
You read ^[\(bookCount) book](inflect: true) this year!
""")
Button("Add a book") {
bookCount += 1
}
}
}
}
In this example, tapping the button increases the bookCount
value, and the text updates automatically. When bookCount
is 1, it uses the singular form book
. As the value increases, such as to 2 or higher, the text dynamically switches to the plural form, ensuring grammatical correctness.
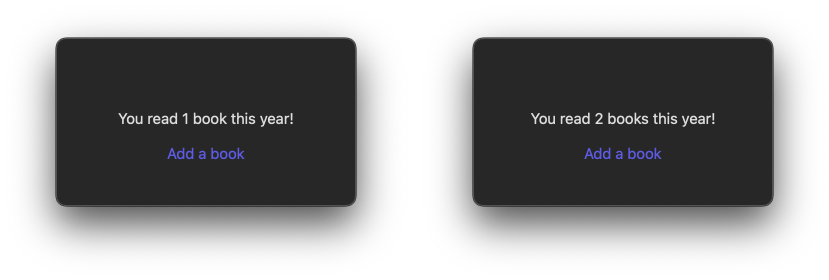
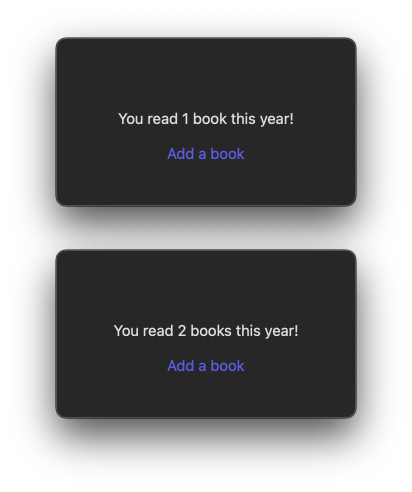
This integration makes handling plurals in SwiftUI both simple and efficient. The automatic grammar agreement feature and the inflection attribute were introduced in iOS 15 with initial support for English and Spanish. Over the years, the grammar engine was expanded to include additional languages, and as of iOS 18, it also supports German, French, Italian, Portuguese, Hindi, and Korean, making it even more versatile for multilingual apps.
If you want to build a strong foundation in SwiftUI, my new book SwiftUI Fundamentals takes a deep dive into the framework’s core principles and APIs to help you understand how it works under the hood and how to use it effectively in your projects.
For more resources on Swift and SwiftUI, check out my other books and book bundles.