Access colors and images from asset catalog via static properties in Xcode 15
Xcode 15 came with a great new way to access colors and images stored in asset catalog. It automatically generates static properties corresponding to our assets on new ColorResource
and ImageResource
types. We can then use those properties to initialize colors and images in SwiftUI, UIKit and AppKit where we previously had to use string literals.
We are going to look at some SwiftUI examples more closely, but keep in mind that equivalent APIs are available in UIKit and AppKit as well.
Let's imagine that we have a custom color called "MyGreen" stored in the asset catalog.
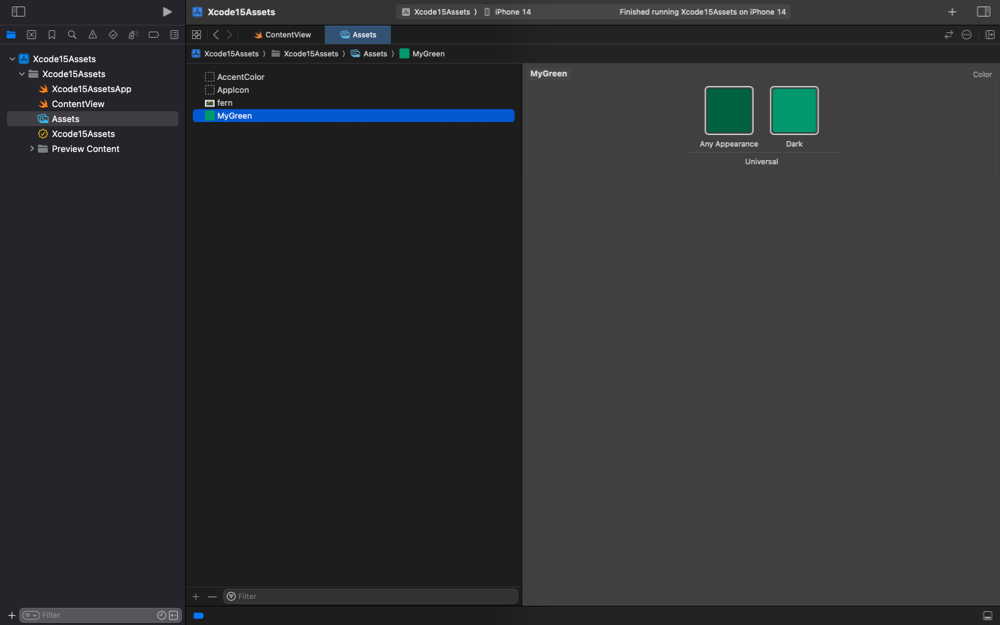
To reference this color in our SwiftUI code, we simply need to call the new Color
initializer that accepts a ColorResource
and pass it .myGreen
.
struct ContentView: View {
var body: some View {
Color(.myGreen)
}
}
The static property myGreen
was created automatically by Xcode, all we needed to do is to define the color in the asset catalog. And it works with autocomplete too. No more string literals to reference the resources 🎉
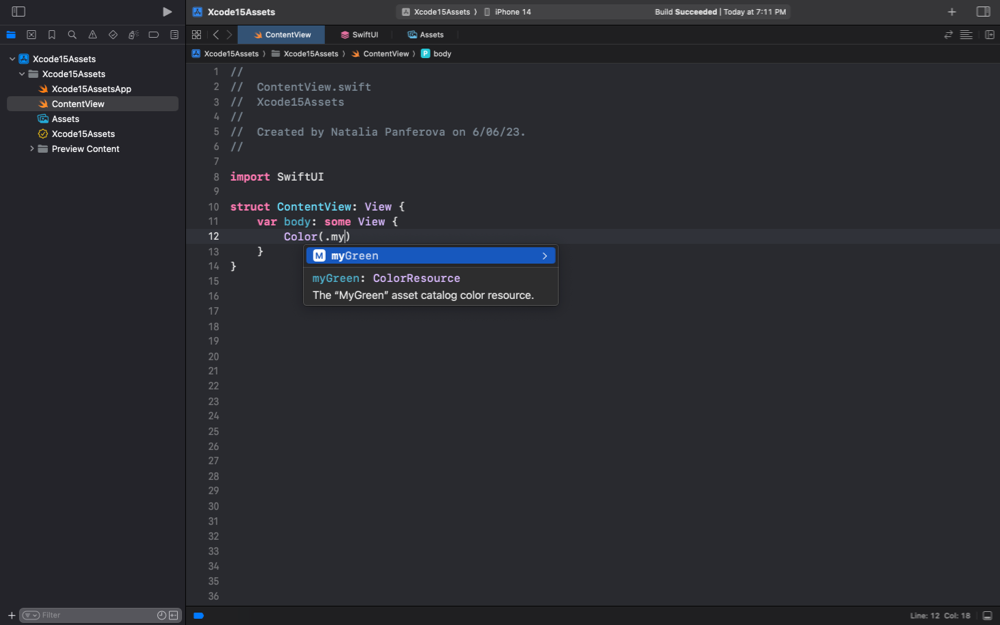
The same applies to image assets too. Let's say we are storing an image called "fern" in the asset catalog.
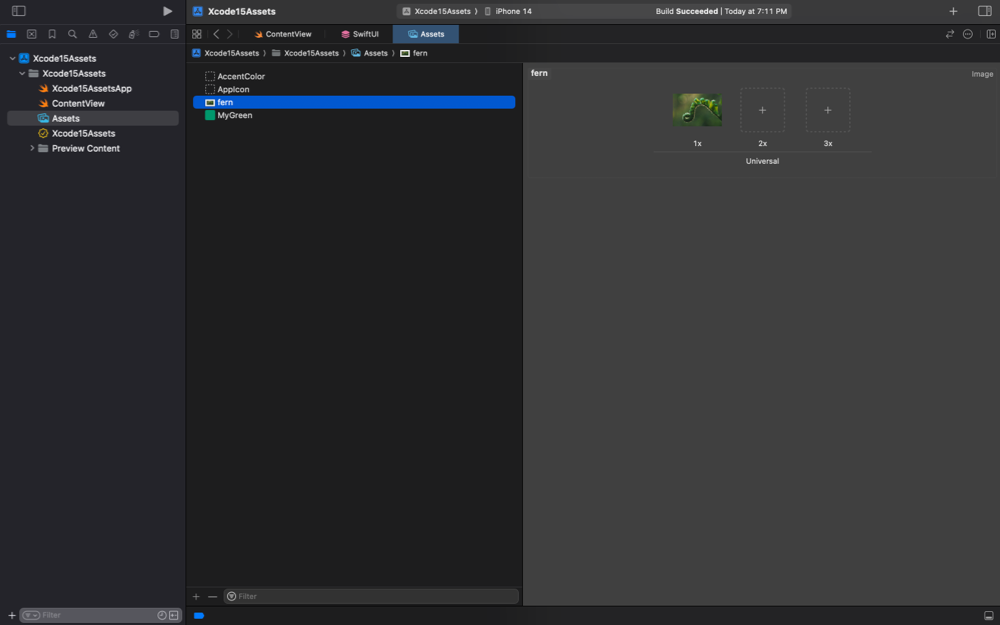
To create a SwiftUI image that shows the fern from the asset catalog, we'll call the new Image
init that accepts an ImageResource
and pass it .fern
.
struct ContentView: View {
var body: some View {
Image(.fern)
}
}
# Generate Swift Asset Symbol Extensions setting
Xcode 15 takes this feature even further with the “Generate Swift Asset Symbol Extensions” setting that is enabled by default, but can be disabled by us if needed.
We can access our assets via static properties generated directly on system types, such as Color
. So we could reference our custom green color from the asset catalog as follows.
struct ContentView: View {
var body: some View {
Color.myGreen
}
}
Note, that as of Xcode 15 beta 1, this doesn't work on the SwiftUI Image
type, referencing Image.fern
will not compile.
If you would like to check out how this setting is enabled or need to disable it in your project, you can search for ASSETCATALOG_COMPILER_GENERATE_SWIFT_ASSET_SYMBOL_EXTENSIONS
in build settings in Xcode.
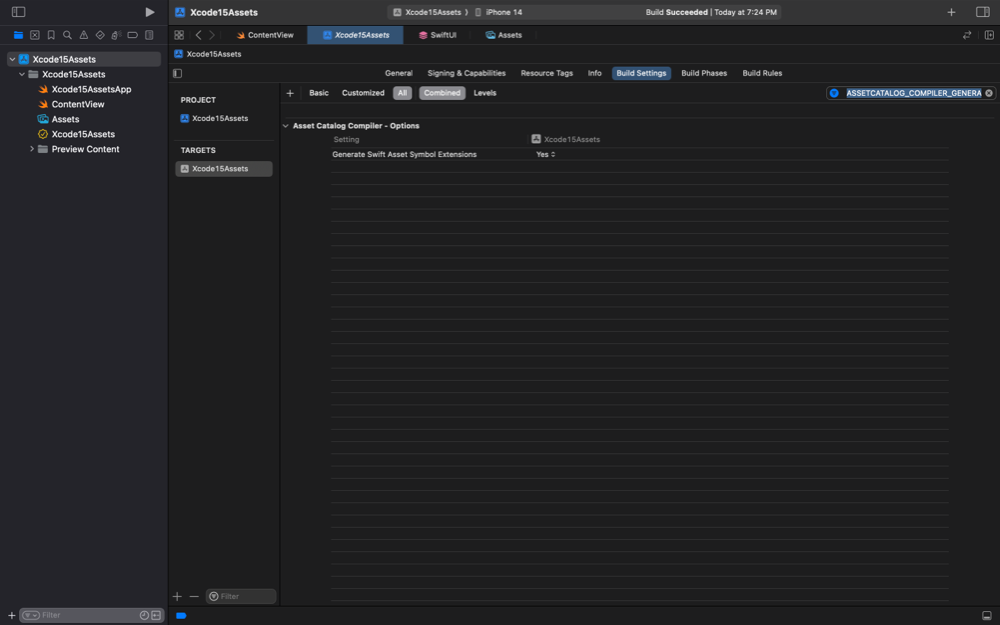
I think this new Xcode 15 behavior is a huge improvement over accessing our asset catalog resources via string literals, like we had to do previously. Our code will now be safer and cleaner.
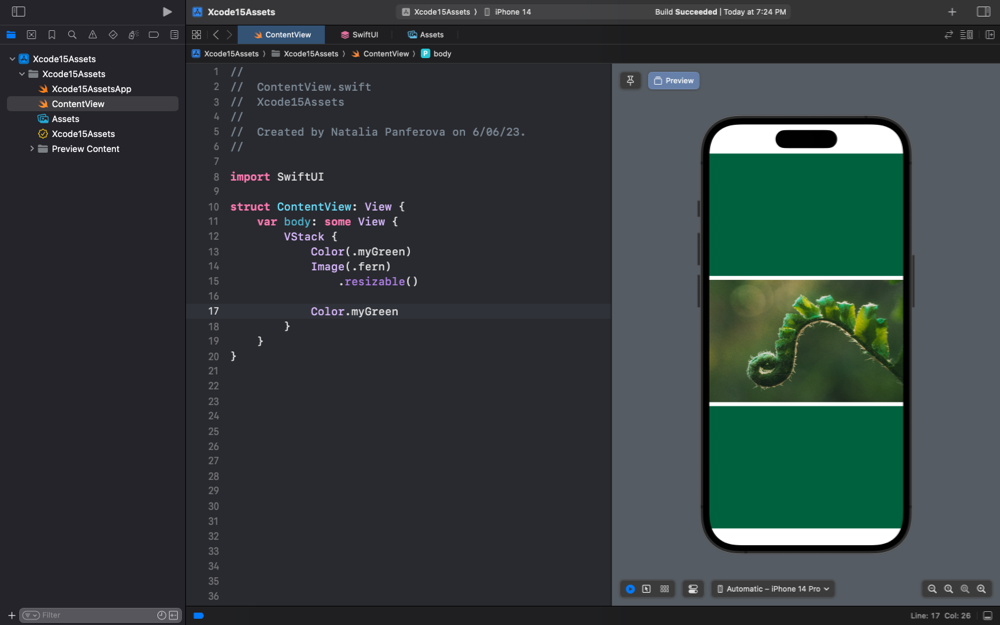
And the greatest thing about this feature is that it works on older deployment targets too, not just iOS 17!
If you're an experienced Swift developer looking to learn advanced techniques, check out my book Swift Gems. It’s packed with tips and tricks focused solely on the Swift language and Swift Standard Library. From optimizing collections and handling strings to mastering asynchronous programming and debugging, "Swift Gems" provides practical advice that will elevate your Swift development skills to the next level. Grab your copy and let's explore these advanced techniques together.