Check localizable strings with the accented pseudolanguage in Xcode
There are a few different ways to declare localizable strings in a SwiftUI app, such as create a Text
view with a string literal, explicitly declare a LocalizedStringKey
variable or initialize a string with the String(localized:)
API from Foundation. But if we are not careful we can miss out on localization of some strings in the app. This can happen, for example, when we create a Text
view with a string variable and want that string to be localizable.
Let's imagine that we are working with the following UI where we have three different strings and we want to localize all of them. At the moment though, the parkDescription
string won't be localized, because it's a regular Swift string, not a LocalizedStringKey
.
struct ContentView: View {
let parkDescription = """
A national park in the heart of
the Southern Alps/Kā Tiritiri o te Moana
"""
let historyNote = String(
localized: """
Arthur's Pass was the first national park
in the South Island of New Zealand.
"""
)
var body: some View {
VStack(alignment: .leading, spacing: 10) {
Text("Arthur's Pass")
.font(.headline)
Text(parkDescription)
Text(historyNote)
.font(.footnote)
.foregroundColor(.secondary)
}
.padding(10)
}
}
To make it easy to spot such strings in the app that won't be localized by default, we can use the accented pseudolanguage in Xcode. By previewing our UI in the accented pseudolanguage we can notice the strings we missed and make them localizable.
We can enable the pseudolanguage from the scheme settings in Product > Scheme > Edit Scheme. In the Options tab of the Run scheme we need to replace the App Language by the Accented PseudoLanguage that can be found at the very end of the language options list among other pseudolanguages.
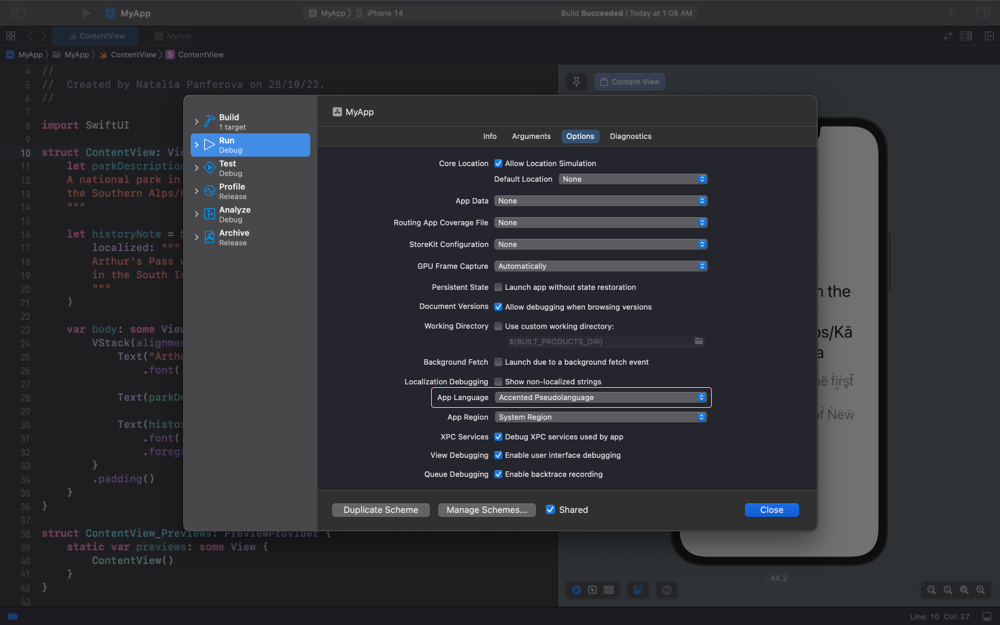
We can now run the app on a device or simply check the view in Xcode previews and see which strings will be localized and which strings are missing. The localizable strings will get the accents in contrast to the non-localizable ones.
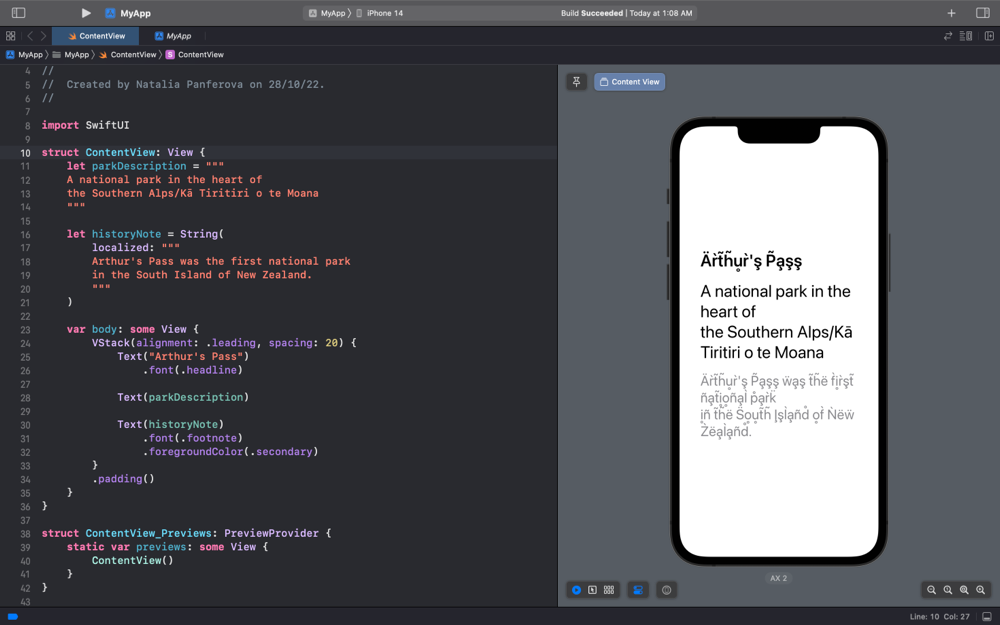
Once we spotted the missing localizations, we can go back to code and fix them, so that all of the strings that need to be localized in the app get exported and translated properly. One of the ways to fix the missing localization is to declare the string variable as a LocalizedStringKey
, which we can do by specifying its type explicitly.
let parkDescription: LocalizedStringKey = """
A national park in the heart of
the Southern Alps/Kā Tiritiri o te Moana
"""
If you would like to learn more about how the Text
view works in SwiftUI and why it automatically localizes strings in some situations and doesn't in others, you can watch my talk from iOSDevUK called Mysteries of SwiftUI Text View.