Keyboard driven navigation with focusable() on iPad in SwiftUI
The recent updates to iOS 17 and iPadOS 17 have brought about exciting changes, one of which is the introduction of the focusable() modifier. This allows us to let users focus on views that are not focusable by default, significantly improving keyboard based interaction.
Here's a simple example of how we can use the focusable()
modifier in SwiftUI.
VStack {
FirstView()
.focusable()
SecondView()
.focusable()
}
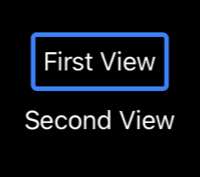
# Customize focus effect style
To customize the style of the focus effect we need to remove the default styling with focusEffectDisabled() and then apply our own.
To know that a view is focused we need to use the focused() modifier with a @FocusState to track and respond to changes.
struct ContentView: View {
var body: some View {
VStack {
FirstViewWrapped()
.focusEffectDisabled()
SecondView()
.focusable()
}
}
}
struct FirstViewWrapped: View {
@FocusState var isFocused
var body: some View {
FirstView()
.focusable() // Enable focuses
.focused($isFocused) // Detect focuses
.background {
if isFocused {
Capsule()
.fill(.indigo)
.opacity(0.3)
}
}
}
}
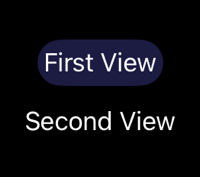
The order of the focusable()
and focused()
modifiers is important. The focused()
modifier needs to be placed after the focusable()
modifier.