Text concatenation vs Text interpolation in SwiftUI
SwiftUI allows us to combine multiple Text
views into a single view using the plus (+
) operator. This enables us to apply different styles to individual parts of the text.
Here's a simple example that colors the word "spicy" in red:
Text("Tortilla chips with ") +
Text("spicy 🌶️🌶️🌶️").foregroundStyle(.red) +
Text(" dip")
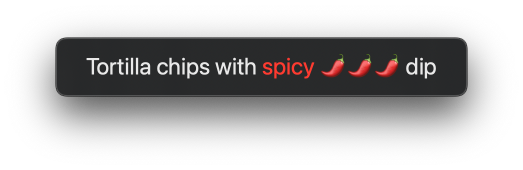
I often see developers using this technique, but it's important to understand its limitations, especially regarding localization.
When we concatenate text like in the example above, each segment will be extracted and translated separately. Here's how it might look in a String Catalog file for French:
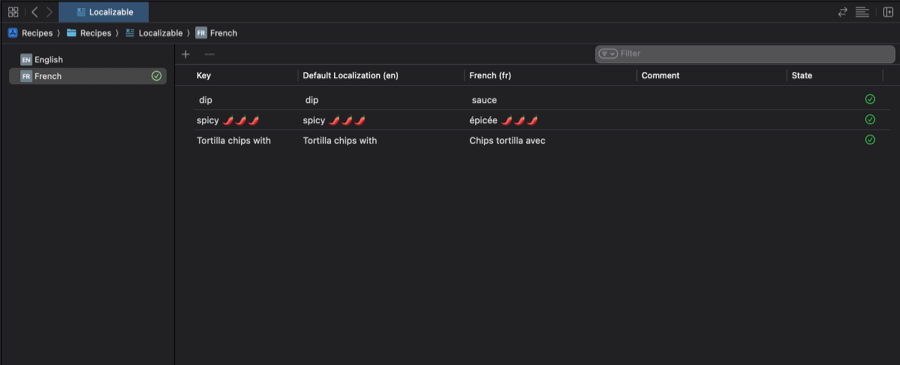
Firstly, some segments have trailing and preceding spaces, which can be quite difficult to spot. Translators must carefully preserve these spaces to ensure that when the translated segments are combined, words still have spaces between them. But there's also a larger issue: concatenation doesn't allow translators to reorder the segments for languages with different grammatical structures. For example, in French, adjectives usually follow the noun, unlike English. The correct translation would be "Chips tortilla avec sauce épicée 🌶️🌶️🌶️", but with simple concatenation, the app would incorrectly display "Chips tortilla avec épicée 🌶️🌶️🌶️ sauce", reflecting a direct translation from English that sounds unnatural.
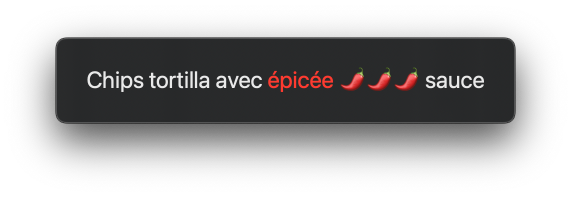
To handle localization properly, we should use text interpolation rather than concatenation. Text interpolation lets us insert variables directly inside a single localizable string, even if these variables are styled Text
views. This means we can apply text modifiers exactly like we do with concatenated segments.
Here's how the previous example looks using interpolation:
Text("Tortilla chips with \(Text("spicy 🌶️🌶️🌶️").foregroundStyle(.red)) dip")
Visually, this renders exactly the same in English:
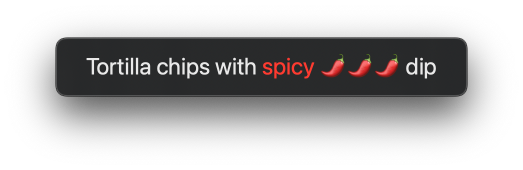
However, the extracted localizable strings in the String Catalog will be very different. While the styled segment is still extracted separately, the main localized string includes a format specifier, allowing translators to reposition the interpolated segment freely within the sentence structure. Additionally, translators no longer need to manage leading and trailing spaces for segments manually.
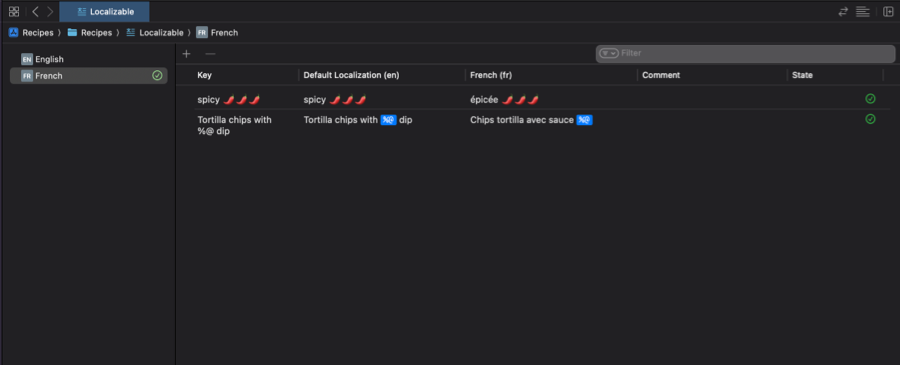
Now, when the app runs in French, SwiftUI correctly inserts the styled adjective into the appropriate place:
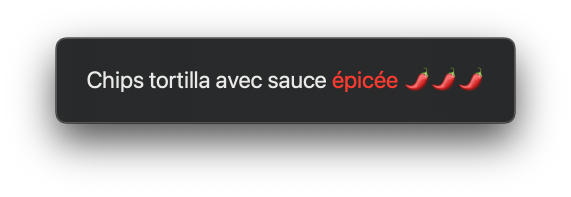
In summary, while text concatenation works well for simple styling scenarios, it's best practice to always prefer interpolation for localized text to ensure grammatically correct and natural translations. In real projects, it's also recommended to include comments, especially for strings with format specifiers, so translators understand what each placeholder represents.
If you want to build a strong foundation in SwiftUI, my new book SwiftUI Fundamentals takes a deep dive into the framework’s core principles and APIs to help you understand how it works under the hood and how to use it effectively in your projects.
For more resources on Swift and SwiftUI, check out my other books and book bundles.